10月28日
农历
十月初四

- 北京市
12
°
55
良
-
多云
- 东南风
- 2级
今天:
晴
9
-
14
℃
- 紫外线 强
- 湿度 53 %
- 日出 06:38
- 日落 17:18
7日内天气预报
-
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级 -
今天 10月28日
多云
12 - 12 ℃东南风 <3级
案例-天气预报#
查询地区天气,数据回显页面
步骤:
- 获取北京市天气数据,展示
- 搜索城市列表,展示
- 点击城市,显示对应天气信息
基本结构#
// 获取并渲染城市天气函数
function getWeather(cityCode) {
// 1.1获取北京市天气数据
myAxios({
url: 'http://hmajax.itheima.net/api/weather',
params: {
city: cityCode
}
}).then(res => {
console.log(res);
...
}).catch(err => {
console.log(err);
})
}
// 默认进入网页就获取天气数据,北京市城市编码:'110100'
getWeather('110100')
请求到的天气数据:
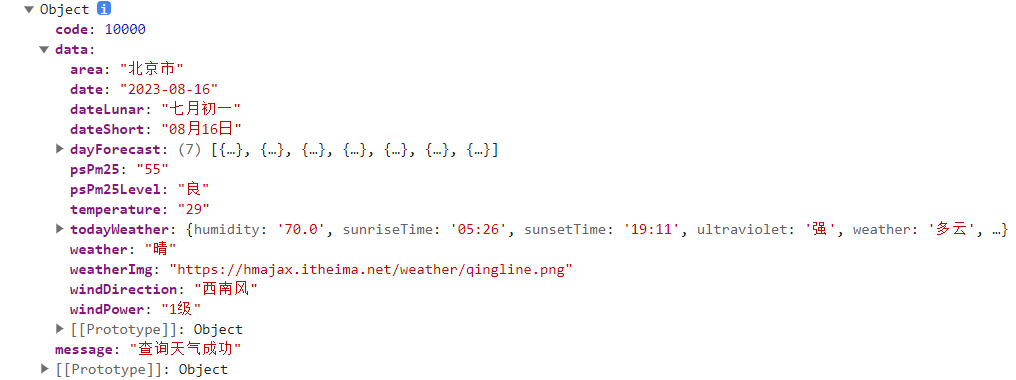
渲染数据#
// 采用模板字符串的方式渲染
({
...
}).then(res => {
console.log(res);
const wObj = res.data;
// 1.2数据回显
// 阳历和农历日期
const dateStr = `<span class="dateShort">${wObj.date}</span>
<span class="calendar">农历
<span class="dateLunar">${wObj.dateLunar}</span>
</span>`
document.querySelector('.title').innerHTML = dateStr;
// 城市名字
document.querySelector('.area').innerHTML = wObj.area;
// 当天气温和当天天气同理,直接采用模板字符串方式渲染
})
7日内天气预报数据渲染
由于7日内天气内容结构一致,天气数据为一个对象数组,所以采用map映射数组的方式来渲染
// 7日内天气预报
const dayForecast = wObj.dayForecast
const dayForecastStr = dayForecast.map(item => {
return `<li class="item">
<div class="date-box">
<span class="dateFormat">${item.dateFormat}</span>
<span class="date">${item.date}</span>
</div>
<img src="${item.weatherImg}" alt="" class="weatherImg">
<span class="weather">${item.weather}</span>
<div class="temp">
<span class="temNight">${item.temNight}</span>-
<span class="temDay">${item.temDay}</span>
<span>℃</span>
</div>
<div class="wind">
<span class="windDirection">${item.windDirection}</span>
<span class="windPower">${item.windPower}</span>
</div>
</li>`
})
document.querySelector('.week-wrap').innerHTML = dayForecastStr
搜索城市列表#
需求:根据关键字,展示匹配城市列表
步骤:
- 绑定input事件,获取关键字
- 获取展示城市列表数据
// 2.1 input获取关键字
document.querySelector('.search-city').addEventListener('input', function(e) {
console.log(e.target.value);
// 2.2 获取城市列表
myAxios({
url: 'http://hmajax.itheima.net/api/weather/city',
params: {
city: e.target.value
}
}).then(res => {
console.log(res.data);
const citieStr = res.data.map(item => {
return `${item.name} `
}).join('')
document.querySelector('.search-list').innerHTML = citieStr
})
})
展示城市天气#
需求:展示用户搜索查看的城市天气
步骤:
- 检测搜索列表点击事件,获取城市code值
- 复用获取展示城市天气函数
// 3.1事件委托点击获取城市code
document.querySelector('.search-list').addEventListener('click', function(e) {
console.log(e.target);
if (e.target.classList.contains('city-item')) {
const cityCode = e.target.dataset.code
console.log(cityCode);
// 3.2调用获取天气函数
getWeather(cityCode)
}
})